Java Program Based on Array
In this program, you'll learn different techniques ,to print the elements of a given array in Java.
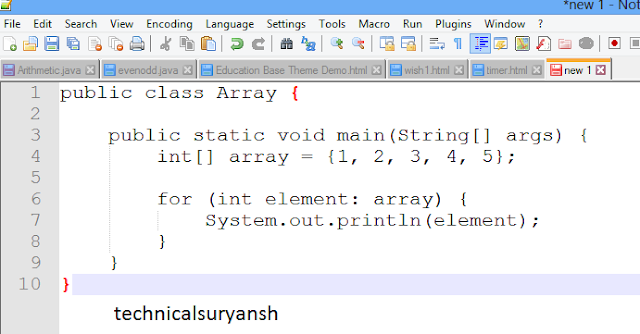
Example 1: Print an Array using For loop
public class Array {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
for (int element: array) {
System.out.println(element);
}
}
}
When you run the program, the output will be:
1 2 3 4 5
Example 2: Print an Array using standard library Arrays
import java.util.Arrays;
public class Array {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(array));
}
}
When you run the program, the output will be:
[1, 2, 3, 4, 5]
Example 3: Print a Multi-dimenstional Array
import java.util.Arrays;
public class Array {
public static void main(String[] args) {
int[][] array = {{1, 2}, {3, 4}, {5, 6, 7}};
System.out.println(Arrays.deepToString(array));
}
}
When you run the program, the output will be:
[[1, 2], [3, 4], [5, 6, 7]]
Check out these related programs:
No comments:
Post a Comment